Check if 2 Strings are Anagram or not
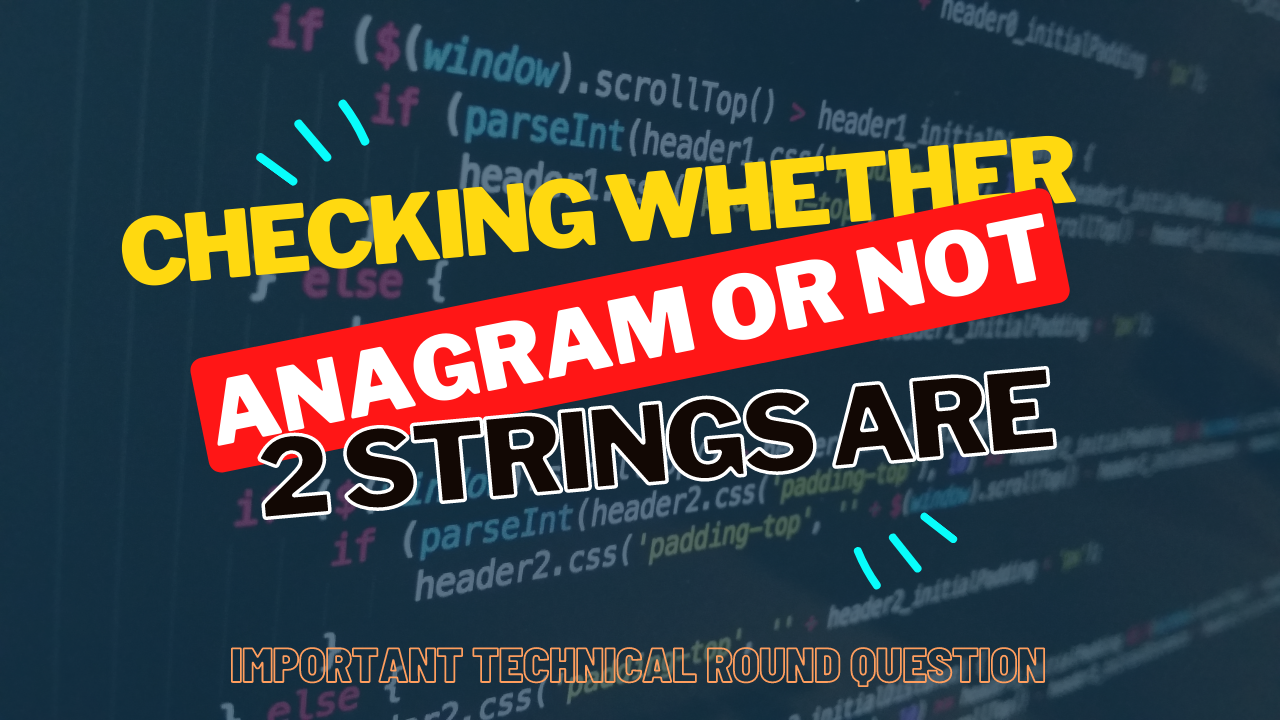
Lets see how we can check whether two strings are anagram or not. Before diving into, lets break the title.
What does Anagram String Mean ?
Lets see with an example. Let, first string be mastr and second string be amtsr. Then this two strings are anagram.
So, we got that Anagram strings are "Strings that are made up of same characters but characters in different sequence."
We will use sorting method to do it. So then for it we will
- sort strings
- compare sorted strings
The source code is here.
1import java.util.Arrays;
2import java.util.Scanner;
3
4public class CheckAnagram {
5
6 public static void main(String[] args) {
7 /*
8 Checking whether two strings are anagram or not.
9 hello
10 lolhe
11
12 Sorting:
13 -> sort strings
14 -> compare sorted strings
15 */
16 // Take 2 string inputs
17 Scanner scanner = new Scanner(System.in);
18 System.out.println("Enter 2 strings:");
19 String str1 = scanner.next();
20 String str2 = scanner.next();
21 scanner.close();
22
23 // convert strings to character arrays for sorting
24 char [] arr1 = str1.toCharArray();
25 char [] arr2 = str2.toCharArray();
26
27 // sorting character arrays in alphabetical order.
28 Arrays.sort(arr1);
29 Arrays.sort(arr2);
30
31 // check and display whether strings are anagram or not.
32 if (checkAnagram(arr1, arr2)) {
33 System.out.println("Strings are anagram.");
34 }
35 else {
36 System.out.println("Strings are not anagram.");
37 }
38 }
39
40 //checkAnagram method to check
41 static boolean checkAnagram(char[] str1, char[] str2){
42 // if length of strings / character arrays are not equal strings will never be anagram
43 if (str1.length != str2.length){
44 return false;
45 }
46 // check each index of both arrays, will never be anagram if each index of both strings have different character
47 for (int i = 0; i < str1.length; i++) {
48 if (str1[i] != str2[i]){
49 return false;
50 }
51 }
52 return true;
53 }
54}
java
THank you for reading. Watch video to be more clear.